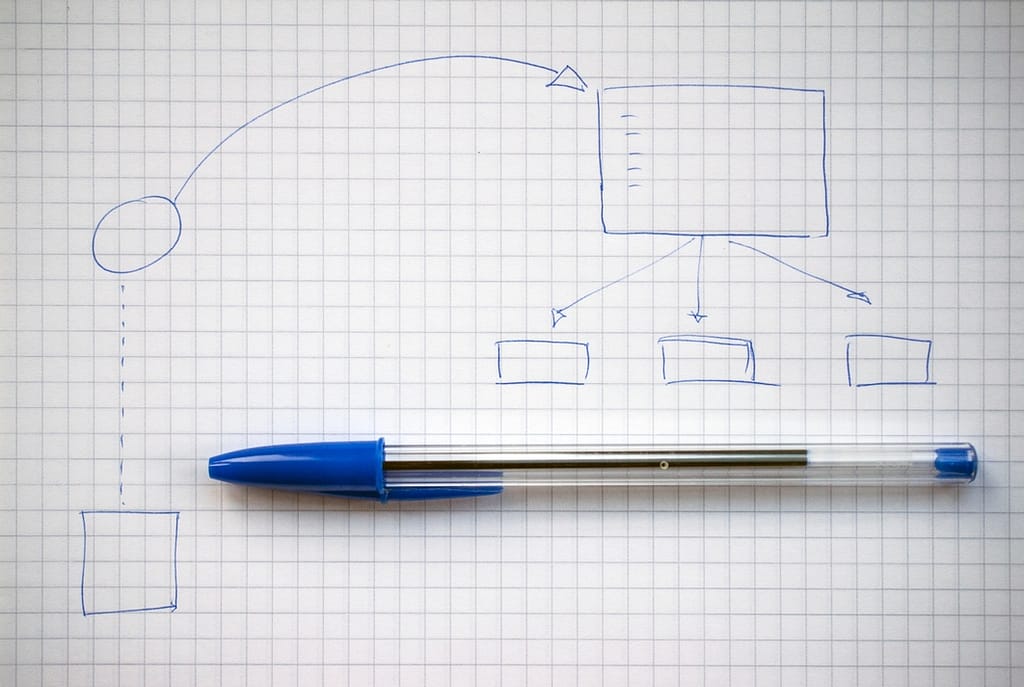
Greetings, Future Tech Trailblazers!
Welcome back to another electrifying episode of “From Detention to Development”! It’s your favorite principal-turned-code-wrangler, ready to unravel the mysteries of the Model-View-ViewModel (MVVM) architecture, another chapter in the Codecademy Ios Developer Career Path. Buckle up, because we’re about to transform our coding skills faster than a teenager’s mood swings!
The Architectural Blueprint: More Than Just a Fancy Floor Plan
Why We Need Design Architectures in Our Digital School
Before we dive into MVVM, let’s chat about why we even need these fancy “design architectures” in our mobile app development classroom.
Imagine if our school had no layout – just a jumble of rooms with no rhyme or reason. Chaos, right? That’s what our code looks like without a proper architecture. These structural blueprints are like our school’s floor plan, guiding us on how to organize our code-classrooms for maximum learning (and minimal confusion).
In the iOS development world, we’ve got a few popular architectural patterns:
- MVC (Model-View-Controller): The old-school approach, like those classic chalkboards.
- MVVM (Model-View-ViewModel): The interactive whiteboard of architectures – modern and efficient.
- VIPER (View, Interactor, Presenter, Entity, Router): The high-tech smart board – powerful but complex.
- Clean Architecture: The whole-school approach to organization – independent and adaptable.
Today, we’re focusing on MVVM – it’s like the Goldilocks of architectures, not too simple, not too complex, but just right for many iOS projects.
MVVM: The Three Musketeers of Code
Let’s break down the MVVM gang – they’re like the dream team of your school staff:
Model: The Librarian
The Model is our data librarian. It knows where all the information is stored, how to retrieve it, and how to process it. It doesn’t care about how the data is displayed – that’s not its department.
Here’s what our Model librarian might look like in Swift:
struct Student {
let id: String
let name: String
let grade: Int
func isHonorRoll() -> Bool {
return grade >= 90
}
}
View: The School Play Director
The View is like our school play director. Its job is to make everything look good on stage (or screen) and capture the audience’s (user’s) reactions. In SwiftUI, it’s the star of the show:
struct StudentProfileView: View {
@ObservedObject var viewModel: StudentProfileViewModel
var body: some View {
VStack {
Text(viewModel.name)
.font(.title)
Text("Grade: \(viewModel.grade)")
.font(.subheadline)
}
}
}
ViewModel: The Vice Principal
The ViewModel is our trusty vice principal, the middleman between the data librarian (Model) and the play director (View). It takes the raw data from the Model, processes it into a format the View can understand, and handles all the behind-the-scenes logic.
class StudentProfileViewModel: ObservableObject {
@Published private var student: Student
var name: String {
return student.name
}
var grade: Int {
return student.grade
}
var isHonorStudent: Bool {
return student.isHonorRoll()
}
init(student: Student) {
self.student = student
}
}
The MVVM School in Action
Now, let’s see how our MVVM school operates:
- The Model (librarian) holds all the student data and core functionality.
- The ViewModel (vice principal) fetches data from the Model, processes it, and makes it ready for display.
- The View (play director) observes the ViewModel and updates the “stage” (UI) when the data changes.
It’s like a well-oiled school machine!
Implementing MVVM in Our “Code History” App
In our Code History app (our digital field trip through programming history), I’ve applied MVVM principles to give it a makeover:
- Project Reorganization: I’ve tidied up our project folders like it’s the first day of school.
- New Model Creation: I’ve created new models to represent our quiz questions and answers – they’re like our digital textbooks.
- New View Creation: I’ve implemented new views to support additional features – think of them as new interactive exhibits in our Code History museum.
- ViewModel Implementation: I’ve created ViewModels to be the tour guides, preparing the exhibit information for our visitors (users) and handling their interactions.
The Honor Roll of MVVM Benefits
Implementing MVVM in your iOS applications is like winning the school science fair:
- Separation of Concerns: Each component has its own job, like a well-organized school staff.
- Testability: We can test our ViewModels independently, like practicing a school play without the costumes.
- Reusability: ViewModels can often be reused, like lesson plans that work for multiple classes.
- Scalability: As our app grows, MVVM provides a clear structure, like adding new classrooms to our school.
- Maintainability: The clear separation makes it easier to update or replace individual components, like swapping out old textbooks for new ones.
The Pop Quiz: Challenges and Considerations
Of course, MVVM isn’t without its challenges:
- Learning Curve: It can be more complex initially, like learning a new subject.
- Potential for Overengineering: In very simple apps, MVVM might be overkill, like using a graphing calculator for basic addition.
- Boilerplate Code: Implementing MVVM can sometimes lead to writing more setup code, like filling out multiple permission slips for one field trip.
- State Management: As apps grow more complex, managing state across multiple ViewModels can become challenging, like keeping track of all the clubs and activities in a large school.
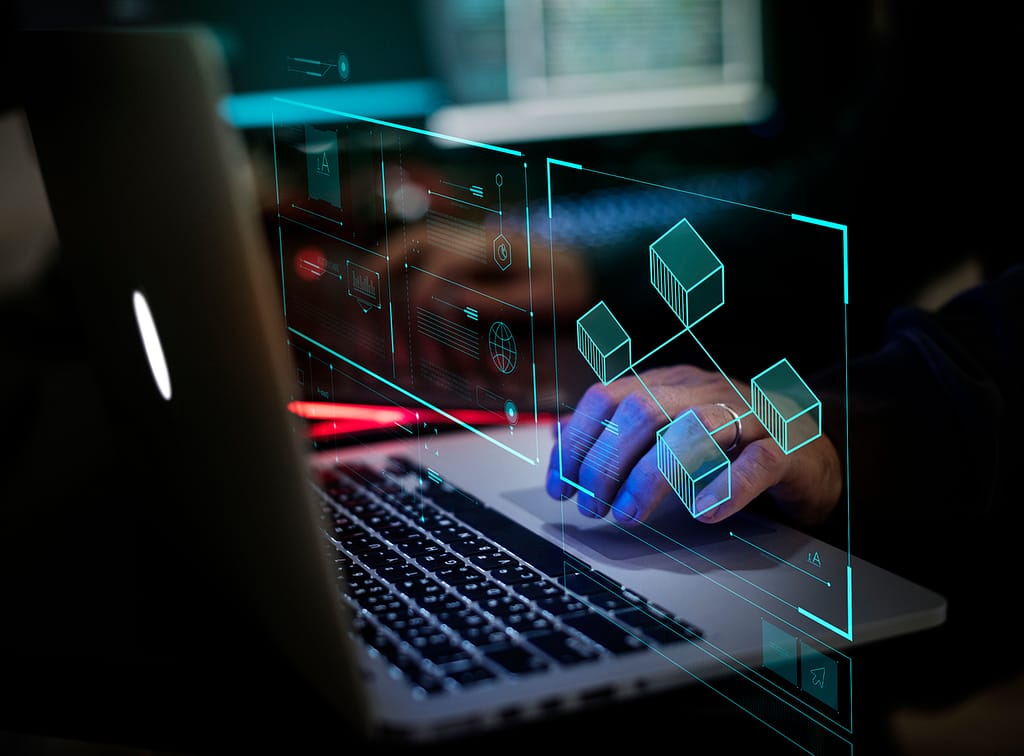
The MVVM Cheat Sheet
Here’s a quick reference table summarizing the key concepts of MVVM:
Concept | Description | Responsibility |
---|---|---|
Model | Represents data and business logic | Data storage, retrieval, and processing |
View | UI components and user interaction | Displaying data, capturing user input |
ViewModel | Intermediary between Model and View | Preparing data for display, handling view logic |
Data Binding | Mechanism for View-ViewModel communication | Keeping View and ViewModel in sync |
Separation of Concerns | Each component has a single responsibility | Improves maintainability and testability |
Testability | Ability to test components in isolation | Particularly beneficial for testing ViewModels |
Scalability | Ease of adding new features | Clear structure for where to add new components |
Graduation Speech
MVVM is like the valedictorian of architectural patterns – it can significantly improve the structure and maintainability of your iOS applications. By clearly separating concerns between Models, Views, and ViewModels, it promotes cleaner, more testable, and more scalable code.
As we continue to develop our Code History app and progress through this iOS development journey, I’m as excited as a kid on the last day of school to see how MVVM will help us create more robust and maintainable applications. Remember, like choosing the right teaching method, MVVM is a tool. The key is understanding when and how to apply it effectively in your projects.
Keep coding, and may your ViewModels always be as organized as a teacher’s lesson plan and your Views as engaging as a school carnival!