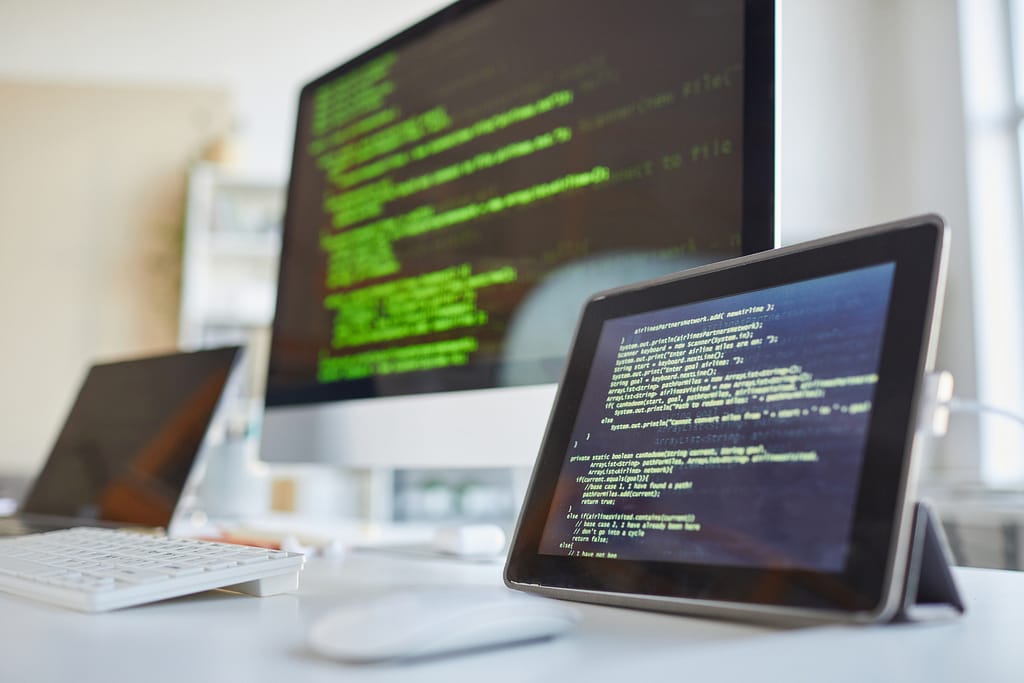
Hello, fellow educators venturing into the world of coding! As a school principal myself, I’ve found that many concepts in Swift programming have surprising parallels to running a school. Today, we’re going to explore enumerations – a powerful tool in Swift that’s as crucial to organized code as a well-structured curriculum is to education. This article comes as always as part of my studying of Codecademy Ios Career Path. So, let’s put on our coding caps and dive in!
What’s an Enumeration, Anyway?
Think of an enumeration (or enum for short) as the departments in your school. Just as you might have “Math”, “Science”, “English”, and “Physical Education” departments, an enum groups related values under a common type. Here’s how we might represent school departments in Swift:
enum SchoolDepartment {
case math
case science
case english
case physicalEducation
}
Now we have a neat package representing all our departments. It’s like having a clear organizational chart for your school – no more confusion about where each subject belongs!
Enums and Switch Statements: As Perfect as a Well-Planned School Day
Enums work beautifully with switch statements, much like how a well-planned school day flows smoothly from one period to the next. Let’s see this in action:
let currentClass = SchoolDepartment.science
switch currentClass {
case .math:
print("Time for calculations!")
case .science:
print("Let's conduct an experiment")
case .english:
print("Open your literature books")
case .physicalEducation:
print("Everyone to the gymnasium")
}
Swift knows all the cases in our enum, so we don’t need a default case. It’s like having a perfect attendance record – every class is accounted for!
Gotta Teach ‘Em All: Iterating Over Enum Cases
Sometimes you need to go through all your departments, perhaps when planning a school-wide event. Swift’s CaseIterable protocol is perfect for this:
enum SchoolDepartment: CaseIterable {
case math, science, english, physicalEducation
}
for department in SchoolDepartment.allCases {
print("Planning activities for: \(department)")
}
This is like effortlessly organizing a curriculum fair where each department gets equal representation.
Raw Values: Giving Your Enum Cases Some Academic Credit
Assigning Raw Values: The Explicit and Implicit Way
Raw values are like assigning credit hours to your courses. Let’s give our departments some numerical codes:
enum SchoolDepartment: Int {
case math = 100
case science = 200
case english = 300
case physicalEducation = 400
}
We’ve explicitly assigned values here. But Swift is as smart as your honor roll students – it can figure out values on its own if we use integers:
enum SchoolDepartment: Int {
case math // implicitly 0
case science // implicitly 1
case english // implicitly 2
case physicalEducation // implicitly 3
}
Extracting Raw Values: The Academic Transcript
Getting the raw value is as easy as checking a student’s transcript:
let mathCode = SchoolDepartment.math.rawValue // 0
print("The code for Math department is: \(mathCode)")
From Raw Value to Enum: The Course Catalog Lookup
We can also go the other way, finding a department from its code:
if let department = SchoolDepartment(rawValue: 2) {
print("Department code 2 is: \(department)") // prints "Department code 2 is: english"
} else {
print("No department with that code!")
}
Remember, all cases in an enum must have the same type of raw value. It’s like ensuring all your courses use the same grading scale for consistency!
Associated Values: When Your Enum Needs to Carry Extra Course Materials
Sometimes, you need your enum cases to carry more information, like how each class might have specific requirements. Let’s upgrade our school system:
enum SchoolClass {
case math(topic: String, difficulty: Int)
case science(experiment: String, safetyGear: [String])
case english(book: String, essayPrompt: String)
case physicalEducation(sport: String, teamSize: Int)
}
let currentClass = SchoolClass.science(experiment: "Volcano Eruption", safetyGear: ["Goggles", "Gloves"])
Now each class carries its own specific details, just like how each subject in school has its unique materials and requirements.
Methods in Enums: Teaching Your Enums New Lessons
Enums can have methods too, just like how each department might have its own teaching strategies:
enum SchoolClass {
case math(topic: String, difficulty: Int)
case science(experiment: String, safetyGear: [String])
case english(book: String, essayPrompt: String)
case physicalEducation(sport: String, teamSize: Int)
func describeClass() -> String {
switch self {
case .math(let topic, let difficulty):
return "Math class on \(topic), difficulty level: \(difficulty)"
case .science(let experiment, let safetyGear):
return "Science class: \(experiment) experiment. Required safety gear: \(safetyGear.joined(separator: ", "))"
case .english(let book, let essayPrompt):
return "English class studying '\(book)'. Essay prompt: \(essayPrompt)"
case .physicalEducation(let sport, let teamSize):
return "PE class playing \(sport) with teams of \(teamSize)"
}
}
}
let todaysClass = SchoolClass.english(book: "To Kill a Mockingbird", essayPrompt: "Discuss the theme of justice")
print(todaysClass.describeClass())
Mutating Methods: When Classes Need a Schedule Change
Sometimes, you might want a method that can change the value of the enum itself. We use the mutating
keyword for this:
enum ClassStatus {
case inSession, breakTime, dismissed
mutating func ringBell() {
switch self {
case .inSession: self = .breakTime
case .breakTime: self = .inSession
case .dismissed: self = .inSession
}
}
}
var currentStatus = ClassStatus.inSession
currentStatus.ringBell()
print(currentStatus) // prints "breakTime"
Computed Properties: Giving Your Enums Some Teacher’s Wisdom
Enums can have computed properties too, which are like experienced teachers who can derive insights from the current situation:
enum Subject {
case math, science, english, history, art
var isSTEM: Bool {
return self == .math || self == .science
}
var recommendedClassSize: Int {
switch self {
case .math, .science: return 20
case .english, .history: return 25
case .art: return 15
}
}
}
let todaysSubject = Subject.science
print("Is this a STEM subject? \(todaysSubject.isSTEM)") // prints "Is this a STEM subject? true"
print("Recommended class size: \(todaysSubject.recommendedClassSize)") // prints "Recommended class size: 20"
These computed properties give us a way to derive values based on the enum case, much like how experienced educators can quickly assess the needs of different classes.
Wrapping It All Up: The Enum Cheat Sheet
Here’s a quick reference table to help you remember all the cool things enums can do:
Feature | Description | Educational Analogy | Example |
---|---|---|---|
Basic Declaration | Define a set of related values | School departments | enum Subject { case math, science, english, history } |
Switch Statement | Exhaustively handle all enum cases | Daily class schedule | switch subject { case .math: print("Time for calculations!") ... } |
CaseIterable | Iterate over all enum cases | School-wide curriculum planning | for subject in Subject.allCases { ... } |
Raw Values | Assign underlying values to cases | Course codes | enum Subject: Int { case math = 100, science = 200, ... } |
Associated Values | Attach additional data to cases | Specific class materials | enum Class { case math(textbook: String, calculator: Bool), ... } |
Methods | Add functionality to enums | Teaching strategies | func describeClass() -> String { ... } |
Mutating Methods | Methods that can change the enum value | Changing class status | mutating func ringBell() { ... } |
Computed Properties | Calculate values based on the enum case | Teacher’s insights | var isSTEM: Bool { return self == .math || self == .science } |
And there you have it, educators! You’re now equipped to use Swift enumerations like a pro. These powerful tools will help you write cleaner, safer, and more expressive code, much like how well-organized school systems lead to better educational outcomes.
Remember, practice makes perfect. For this reason I’m going to incorporate enums into my next coding project and watch my code organization level up, just like how continuous professional development enhances our teaching skills.
Happy coding, and may your enums be as well-organized as your school’s honor roll!