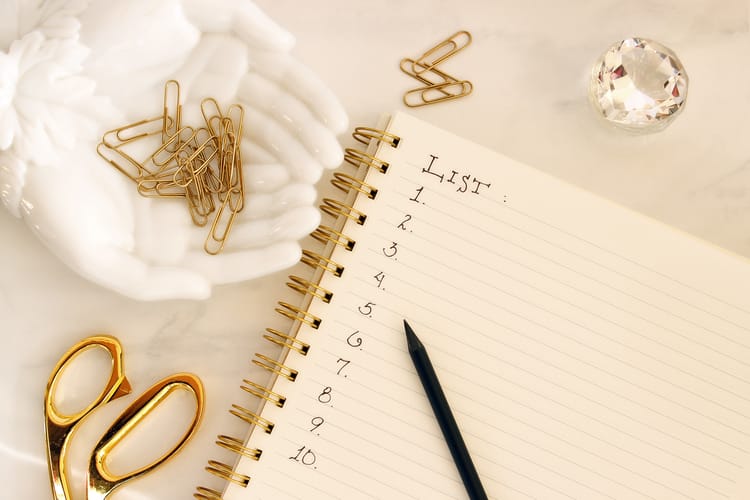
Welcome to the List Party, iOS Developers!
Hello, fellow code enthusiasts! It’s your friendly neighborhood principal-turned-developer here, ready to unravel the mysteries of Swift Lists. As I navigate the treacherous waters of the iOS Developer Career Path from Codecademy, I’ve stumbled upon a game-changer in UI design. So, grab your virtual notepad, and let’s dive into the world of Swift Lists!
What Are Lists, and Why Should We Care?
Imagine you’re organizing the school yearbook. You’ve got a ton of student photos, names, and fun facts to display. In the iOS world, that’s where Lists come in handy. They’re the Swiss Army knife of UI components, perfect for displaying rows of data in a scrollable format.
Lists in SwiftUI are like the cool kids of the UI world – they’re flexible, easy to work with, and they make your app look like it just stepped out of a Silicon Valley fashion show.
Implementing SwiftUI Lists: Static vs Dynamic Data
The Static Approach: When Your Data Doesn’t Dance
Sometimes, your data is as unchanging as the school cafeteria menu. For these cases, we can use a static List. Here’s how it looks:
List {
Text("John Appleseed")
Text("Tim Cook")
Text("Steve Wozniak")
}
Simple, right? It’s like writing names on a chalkboard – straightforward but not very exciting.
The Dynamic Duo: Lists and Collections
Now, let’s spice things up with some dynamic data. Imagine your list of students can change faster than the latest TikTok trend. Here’s how we handle that:
struct Student {
let name: String
}
let students = [
Student(name: "John Appleseed"),
Student(name: "Tim Cook"),
Student(name: "Steve Wozniak")
]
List(students, id: \.name) { student in
Text(student.name)
}
This approach is like having a magical, self-updating yearbook. Pretty cool, huh?
The Identity Crisis: Why Lists Need Identifiable Data
Here’s where things get a bit tricky. SwiftUI Lists are like those strict teachers who need to know exactly who’s who. They require each item to have a unique identifier. It’s like giving each student a unique ID badge.
Method 1: The Keypath Approach
Remember our previous example? We used id: \.name
to tell SwiftUI how to identify each student. It’s like saying, “Hey, SwiftUI, use the student’s name as their ID badge.”
Method 2: The Identifiable Protocol – When Your Data Wants to Introduce Itself
For a more sophisticated approach, we can make our data model conform to the Identifiable
protocol. It’s like teaching our data to shake hands and introduce itself:
struct Student: Identifiable {
let id = UUID()
let name: String
}
let students = [
Student(name: "John Appleseed"),
Student(name: "Tim Cook"),
Student(name: "Steve Wozniak")
]
List(students) { student in
Text(student.name)
}
Now SwiftUI knows exactly how to identify each student without us having to play matchmaker.
ForEach: The List’s Cool Cousin
Sometimes, you want more control over your list items. That’s where ForEach
comes in. It’s like the cool teacher who lets you customize your project:
List {
ForEach(students) { student in
HStack {
Image(systemName: "person.circle")
Text(student.name)
}
}
}
With ForEach
, you can add custom views, apply modifiers, or even add interactivity to each item. It’s the Swiss Army knife within the Swiss Army knife!
Sections: Organizing Your List Like a Pro
Remember how the yearbook is often divided into grades or classes? In SwiftUI, we can do something similar with Section
s:
List {
Section(header: Text("Freshmen")) {
ForEach(freshmenStudents) { student in
Text(student.name)
}
}
Section(header: Text("Sophomores")) {
ForEach(sophomoreStudents) { student in
Text(student.name)
}
}
}
Sections make your lists prettier and easier to navigate. It’s like giving your digital yearbook a table of contents!
Putting It All Together: The Ultimate School Directory
Now, let’s combine everything we’ve learned to create the ultimate school directory:
struct Student: Identifiable {
let id = UUID()
let name: String
let grade: Int
}
struct ContentView: View {
let freshmen = [Student(name: "Alice", grade: 9), Student(name: "Bob", grade: 9)]
let sophomores = [Student(name: "Charlie", grade: 10), Student(name: "David", grade: 10)]
var body: some View {
List {
Section(header: Text("Freshmen")) {
ForEach(freshmen) { student in
HStack {
Image(systemName: "person.circle")
Text(student.name)
Spacer()
Text("Grade: \(student.grade)")
}
}
}
Section(header: Text("Sophomores")) {
ForEach(sophomores) { student in
HStack {
Image(systemName: "person.circle.fill")
Text(student.name)
Spacer()
Text("Grade: \(student.grade)")
}
}
}
}
}
}
And there you have it! A beautiful, organized, and dynamic school directory that would make any principal proud.
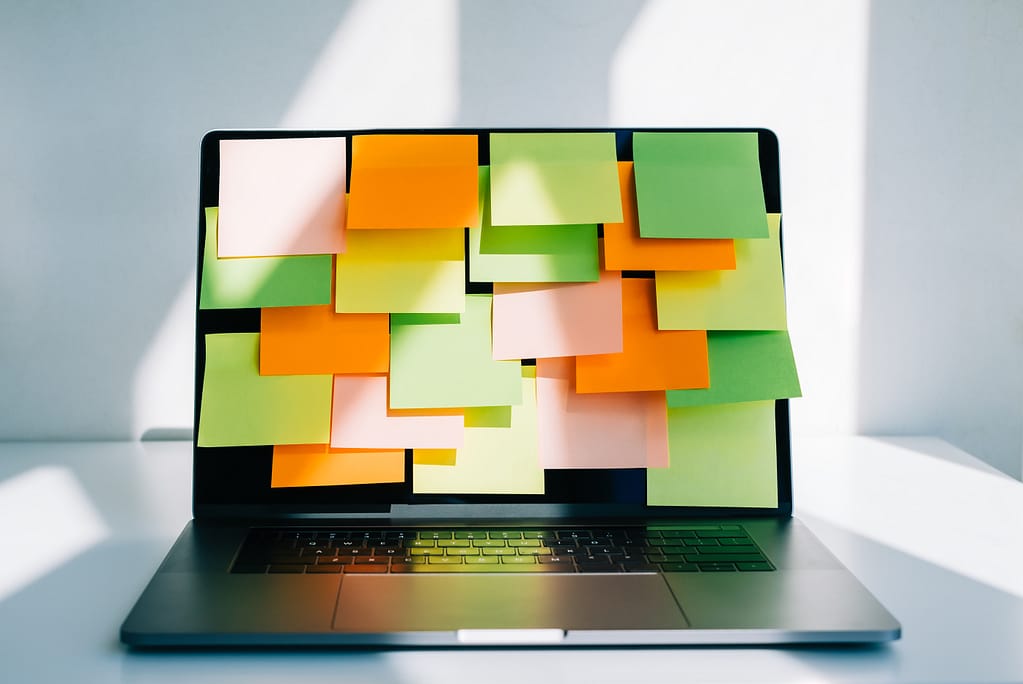
The SwiftUI Lists Cheat Sheet
Here’s a quick reference table to help you remember the key concepts we’ve covered:
Concept | Description | Use Case |
---|---|---|
Static List | List with hardcoded items | Small, unchanging data sets |
Dynamic List | List populated from a collection | Larger or changing data sets |
Identifiable | Protocol for unique item identification | When using List or ForEach |
ForEach | Structure for custom list item views | More control over list item appearance |
Section | Groups list items with optional header/footer | Organizing lists into categories |
Graduation Speech
Congratulations, iOS developers! You’ve just added a powerful tool to your SwiftUI toolkit. Lists are the backbone of many iOS apps, from simple to-do lists to complex social media feeds. By mastering Lists, you’re one step closer to creating those sleek, professional iOS apps you’ve been dreaming about.
Remember, like in education, the key to great app development is organization and clarity. SwiftUI Lists help you achieve both, making your code cleaner and your UIs more intuitive.
Keep coding, keep learning, and may your Lists always be as organized as a well-planned lesson and as dynamic as a classroom full of eager students!